Depending on where you look, the current census on programming languages tells us that there are anywhere from 750 to 10,000 programming languages. Out of which, only around 200 are considered notable. If your goal is to learn to program for the sake of building a career, it pays to know which languages are worth learning.
In this article, we take a deep dive into the world of programming languages to explore the best ones to learn in 2024. We’ll cover each language’s history and its strengths, and we’ll look at things like salaries and their popularity amongst professional developers, as well as highlight use cases and useful resources.
Whether you are looking to start a career in programming or are a seasoned developer, this article provides a comprehensive overview of the best programming languages to help you stay ahead of the curve.
🏆 How are the languages ranked in this list?
This list of the currently most popular programming languages is based on four ranking systems but also considers how in-demand a particular language is on job hunting sites. The ranking systems in question are StackOverflow Developer Survey, PYPL Index, TIOBE Index, and GitHub Activity.
These sites will not be linked again in this article, so you can either check them out in your own time or open them now. A separate table section for Popularity will include the rankings taken from the said sites for each language mentioned in this article.
1. Python
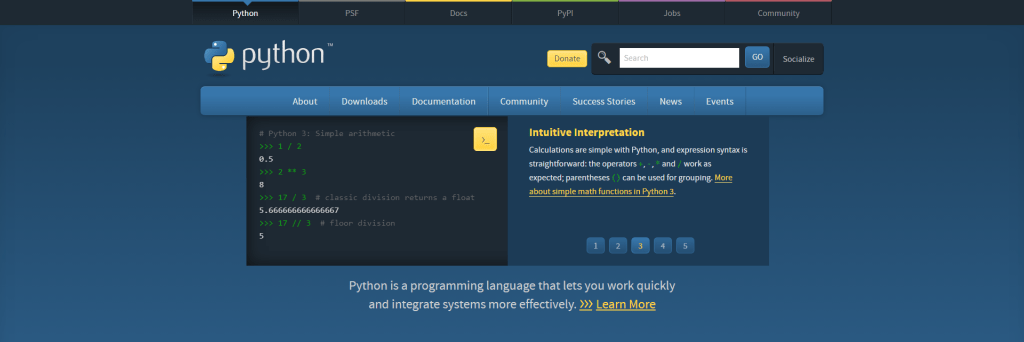
Python is a high-level, general-purpose programming language that has gained popularity among developers of all levels over the years. It is known for its simplicity, readability, and ease of use, making it a great choice for beginners who are just starting out in programming, as well as professionals who need to write code quickly and efficiently.
One of the most attractive features of Python is its readability. Its syntax is easy to understand, which means that even new programmers can write code that is easy to read and maintain. This makes it an excellent choice for beginners, who can focus on learning programming concepts without getting bogged down by complicated syntax.
In addition, Python has a vast and supportive community. There are many resources available to help developers of all levels, including tutorials, documentation, and online forums. This makes it easy to learn and use Python, and to get help when you need it.
Python can be used for a wide range of applications, from web development and data science to machine learning and artificial intelligence. This means that whether you’re a beginner or a professional, you can find a use for Python in your work.
Difficulty | Python is considered to be a relatively easy language to learn and use, particularly for beginners. |
Learning curve | The learning curve for Python can vary depending on your previous programming experience. |
Requirements | Python can run on a variety of platforms, including Windows, macOS, and Linux. It can be run using a number of different IDEs, such as PyCharm, IDLE, or directly from the CMD. |
Versatility (Use cases) | ▸ Web development ▸ Data analysis ▸ Scientific computing ▸ Artificial Intelligence ▸ Scripting and automation ▸ Machine Learning |
Developer Salary | $117,582/year (US) ━ €62,500/year (EU) |
Popularity | ❯ StackOverflow Survey: 65.52% approval rate. ❯ PYPL Index: ranked #1. ❯ TIOBE Index: ranked #1. ❯ GitHub: ranked #2. |
Pros | – Easy to read and write – Large community and ecosystem – Highly versatile |
Cons | – Can be slower than some other languages – Use of dynamic typing can sometimes lead to errors that are only caught at runtime |
Useful links | ▪ Website ▪ Documentation ▪ Awesome Python ▪ Python Hosting ▪ The history of Python |
2. JavaScript
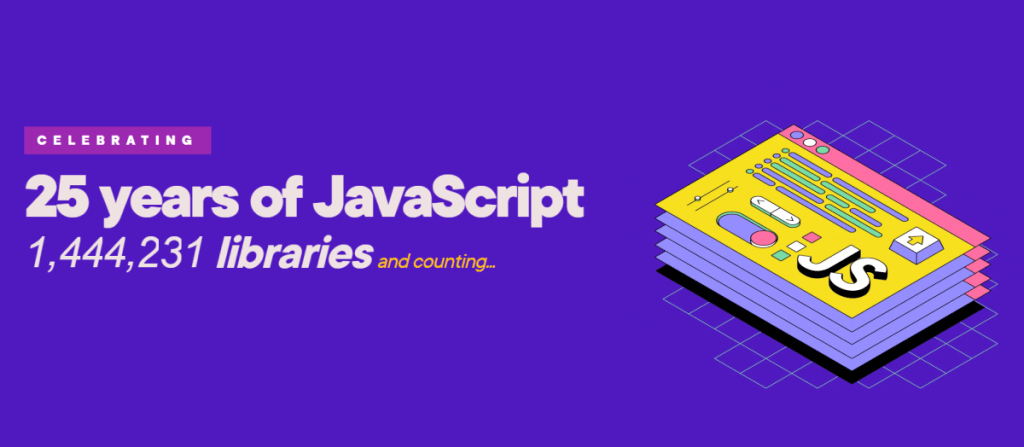
JavaScript is a high-level, dynamic programming language that is known as the go-to language for web development. It’s one of the most widely used programming languages in the world and is supported by all major web browsers.
One of the things that makes JavaScript so popular is its versatility. It can be used for a wide range of applications, from interactive web pages and web applications, to server-side programming and mobile app development. JavaScript is also an excellent choice for building cross-platform mobile apps, using frameworks such as React Native and Ionic.
Another reason for JavaScript’s popularity is its relatively easy-to-learn syntax. This makes it a great language for beginners to start with, while still being powerful enough to handle complex tasks and projects. JavaScript is also constantly evolving, with new features and updates being added regularly. This means that there are always new things to learn and explore.
In addition, JavaScript has a massive community of developers and enthusiasts who are constantly creating new libraries, tools, and frameworks to help make programming with JavaScript even easier and more efficient. This means that there are endless resources available to help you learn and improve your skills.
Difficulty | JavaScript is considered a relatively easy language to learn, especially for those who are already familiar with HTML and CSS. However, as with any programming language, the difficulty level will depend on the complexity of the project you are working on. |
Learning curve | The learning curve for JavaScript is generally considered to be moderate. It is a language that is easy to get started with, but can take some time to master. |
Requirements | JavaScript can be used on any device with a modern web browser. All you need to get started is a text editor and a web browser. There are many integrated development environments (IDEs) available as well. |
Versatility (Use cases) | ▸ Creating interactive user interfaces on websites ▸ Building web applications ▸ Developing mobile applications ▸ Developing server-side applications using Node.js ▸ Creating browser extensions and add-ons ▸ Building games and other interactive content |
Developer Salary | $111,427/year (US) ━ €54,500/year (EU) |
Popularity | ❯ StackOverflow Survey: 57.83% approval rate. ❯ PYPL Index: ranked #3. ❯ TIOBE Index: ranked #6. ❯ GitHub: ranked #1. |
Pros | – Widely used and supported by all major browsers – Easy to learn and get started with – Large community with extensive documentation and resources – Versatile and can be used for a wide range of applications – Supports functional, object-oriented, and procedural programming paradigms |
Cons | – Can be easy to make mistakes and introduce bugs due to its dynamic typing – Browser compatibility issues can be a challenge to work with – Security can be a concern, as JavaScript code can be easily accessed and modified by users – Can be difficult to debug and maintain for large projects – As a dynamically typed language, it may be less performant than statically typed languages in certain scenarios. |
Useful links | ▪ Website ▪ Documentation ▪ Awesome JavaScript ▪ JavaScript IDEs |
3. C#
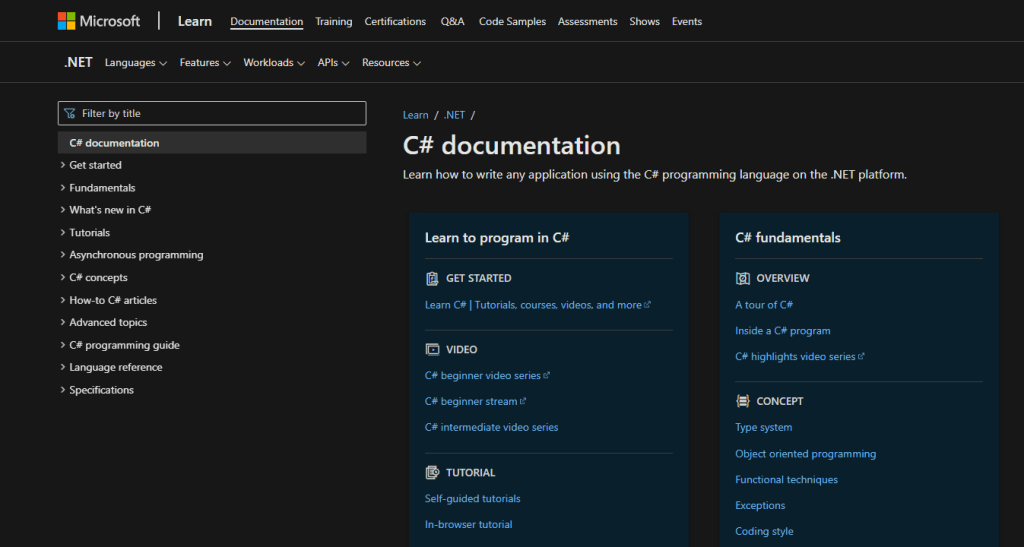
Microsoft developed C# to be powerful and versatile yet accessible to beginners. As a result, it’s a great choice for anyone who wants to learn to code, as well as seasoned professionals who are looking for a new tool to add to their arsenal.
One of the standout features of C# is its versatility. Whether you want to build mobile apps, desktop software, or web applications, C# has you covered. That’s one of the reasons why it’s become such a popular language in recent years, as developers are increasingly looking for a language that can handle a wide range of projects.
But C# isn’t just versatile – it’s also performant. Because it’s a compiled language, the code gets translated into machine code that runs quickly and efficiently. This is particularly important when working on large or complex projects that involve lots of data.
Another thing that sets C# apart is its focus on writing clean, readable code. It supports object-oriented programming, which allows you to write reusable code that can be easily modified and extended over time. C# has a large standard library that comes with a wide range of built-in functions and tools, which can save you time and effort when working on your projects.
Difficulty | C# is considered to be a relatively easy language to learn, especially for developers who are already familiar with other object-oriented programming languages. |
Learning curve | The learning curve for C# is generally considered to be moderate. Developers who are new to programming may need to spend some time getting familiar with basic programming concepts, such as variables, data types, and control structures. However, C# is well-documented, and there are many resources available for developers who are looking to learn the language. |
Requirements | To use C#, you’ll need a computer running the Windows operating system, as C# is a Microsoft language. You’ll also need to download and install the .NET Framework, which is a software platform that provides the runtime environment for C# code to run. There are workarounds for Linux, such as Mono. |
Versatility (Use cases) | ▸ Building desktop applications for Windows ▸ Developing video games and other interactive multimedia applications ▸ Creating web applications using the ASP.NET framework ▸ Building cross-platform mobile applications using Xamarin ▸ Developing enterprise applications and services using .NET Core |
Developer Salary | $101,512/year (US) ━ €55,000/year (EU) |
Popularity | ❯ StackOverflow Survey: 62.87% approval rate. ❯ PYPL Index: ranked #4. ❯ TIOBE Index: ranked #5. ❯ GitHub: ranked #8. |
Pros | – Easy to learn and use for developers with experience in other object-oriented languages – Strong community support and extensive documentation – Well-integrated with other Microsoft technologies, including the .NET Framework and Visual Studio – Designed for building robust, scalable applications – Support for modern programming paradigms, such as functional programming and asynchronous programming |
Cons | – Limited support for non-Windows platforms – Less suitable for low-level system programming compared to languages like C and C++ – Requires significant overhead to get started with a full-featured development environment like Visual Studio – Requires additional runtime dependencies to be installed on client machines to run C# applications. |
Useful links | ▪ Wikipedia ▪ Documentation ▪ Awesome C# |
4. C++
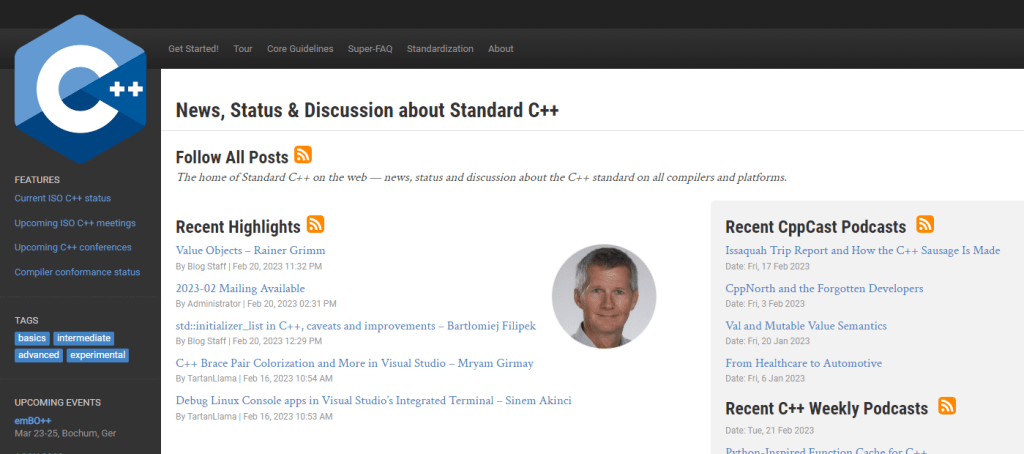
C++ is a powerful programming language that has been used to build some of the most complex and critical software systems in the world. C++ is well-suited for a wide range of applications, from developing video games and operating systems to writing complex algorithms and implementing high-performance scientific simulations.
One of the key strengths of C++ is its performance. C++ was designed to be a high-performance language, allowing developers to write code that runs efficiently and quickly, even on systems with limited resources. This makes it an ideal language for developing software that needs to be highly optimized and responsive, such as video games or real-time systems.
C++ also offers developers a high level of control over their code, allowing them to fine-tune their programs to meet specific performance requirements. This control is possible due to the fact that C++ is a low-level language, which means that it provides direct access to hardware resources and memory management. This allows developers to create highly efficient code, without having to rely on layers of abstraction that can slow down performance.
Despite its performance-oriented design, C++ is also an incredibly flexible and adaptable language. It supports a wide range of programming paradigms, including object-oriented, functional, and generic programming. This means that developers can choose the approach that best fits their needs, and can write code that is both clean and maintainable.
C++ also offers a wealth of libraries and frameworks, making it easy to develop complex software systems without having to write everything from scratch. Additionally, because C++ has been around for over 30 years, there is a vast community of developers who have contributed to its development and are available to provide support and guidance.
Difficulty | C++ can be a difficult language to learn, especially for beginners who are new to programming. |
Learning curve | C++ has a steep learning curve due to its complex syntax and concepts. However, once you become proficient in C++, you can use it to build complex and efficient applications. |
Requirements | C++ can be used on a variety of platforms, including Windows, Linux, and macOS. To use C++, you need a text editor or an Integrated Development Environment (IDE), a compiler, and the necessary libraries. |
Versatility (Use cases) | ▸ Operating systems development ▸ Game development ▸ Web browsers development ▸ High-performance applications ▸ Embedded systems |
Developer Salary | $117,500/year (US) ━ €61,000/year (EU) |
Popularity | ❯ StackOverflow Survey: 49.77% approval rate. ❯ PYPL Index: ranked #5. ❯ TIOBE Index: ranked #3. ❯ GitHub: ranked #5. |
Pros | – C++ is a high-performance language that can be used to build efficient and fast applications. – It allows low-level memory manipulation, which can be useful in certain use cases. – It has a large community and plenty of resources and libraries available. |
Cons | – C++ can be difficult to learn, especially for beginners. – The language is verbose, which can make it harder to write and read code. – C++ is prone to memory leaks and segmentation faults if not handled correctly. |
Useful links | ▪ Website ▪ Documentation ▪ Awesome C++ ▪ C++ IDEs |
5. PHP
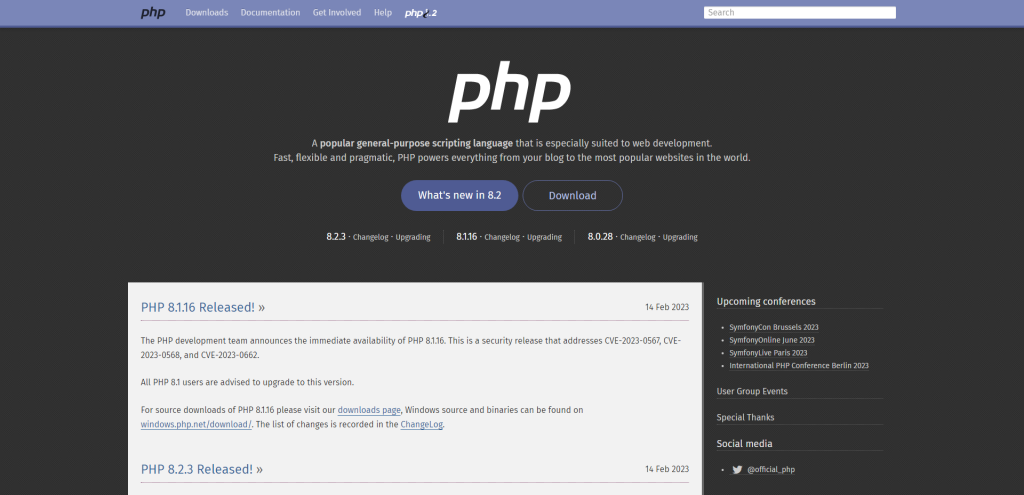
If you’re interested in programming, you may have heard of PHP, one of the most popular programming languages out there. PHP, which stands for “Hypertext Preprocessor,” is a server-side scripting language that’s primarily used for web development. It was first created in 1994 by Rasmus Lerdorf and has since evolved into a powerful, versatile language that both beginners and experienced developers alike use.
At its core, PHP is a server-side scripting language that’s designed to work seamlessly with web servers like Apache and Nginx. This means that you can use PHP to build dynamic web pages that can interact with databases, handle user input, and perform a variety of other tasks that are essential to modern web development.
But perhaps the biggest advantage of PHP is its ability to help you write clean, maintainable code. With features like object-oriented programming, automatic memory management, and a wide range of built-in functions and libraries, PHP makes it easy to create code that’s easy to read, test, and maintain over time.
Difficulty | PHP is generally considered to be a relatively easy language to learn and use, particularly for web development. |
Learning curve | The learning curve for PHP is generally considered to be relatively short, particularly for those with prior programming experience. However, like any language, mastery can take time. |
Requirements | PHP can be run on any web server that supports PHP, which is most servers. Additionally, a text editor or integrated development environment (IDE) is generally needed to write PHP code. |
Versatility (Use cases) | ▸ Building dynamic websites and web applications ▸ Server-side scripting for web development ▸ Command-line scripting ▸ Developing e-commerce platforms and content management systems (CMS) ▸ Creating custom CMS themes and plugins ▸ Building web-based applications that require server-side processing |
Developer Salary | $85,326/year (US) ━ €40,000/year (EU) |
Popularity | ❯ StackOverflow Survey: 41.83% approval rate. ❯ PYPL Index: ranked #6. ❯ TIOBE Index: ranked #8. ❯ GitHub: ranked #9. |
Pros | – Easy to learn and use, particularly for web development – Strong community support and resources – Wide range of built-in functions and libraries for common tasks – Integrates well with other web technologies, such as HTML and CSS – Works well with most popular databases, such as MySQL and PostgreSQL |
Cons | – Can be less performant than other programming languages, particularly for large-scale applications – Not as well-suited for general-purpose programming as some other languages – Some developers criticize PHP for its inconsistent syntax and design choices – Security concerns related to vulnerabilities in the language’s core or in poorly written code. |
Useful links | ▪ Website ▪ Documentation ▪ Awesome PHP ▪ PHP Hosting |
6. Swift
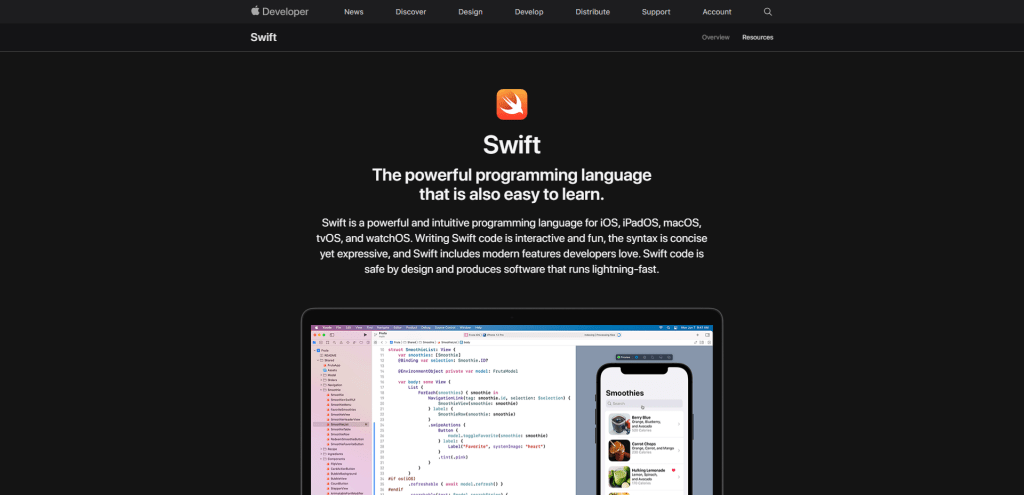
Swift was first introduced by Apple in 2014, with the aim of creating a language that would be easier to read and write than Objective-C, but still offer the high performance and safety that professional developers require. Since then, Swift has experienced massive growth and adaptation by some of the largest organizations in the world.
With Swift, you can write code for iOS, iPadOS, macOS, tvOS, and watchOS, making it incredibly versatile. Furthermore, Swift is designed to be interoperable with Objective-C, which means that you can use it in conjunction with existing code bases, making it an ideal choice for companies with established code.
Swift is incredibly versatile and user-friendly, even for beginners. In fact, it was designed to be anyone’s first programming language, whether you’re still in school or exploring new career paths. Educators can download free curriculum to teach Swift both in and out of the classroom, while first-time coders can use Swift Playgrounds to learn and interact with the language.
Difficulty | Swift is generally considered to be a relatively easy language to learn, especially for beginners who are new to programming. It has a straightforward syntax that is easy to read and write, which can make it easier for developers to create code quickly. |
Learning curve | As mentioned, the learning curve for Swift is relatively gentle compared to some other programming languages. Apple provides extensive documentation and a range of online resources to help developers get started with Swift, which can be useful for those who are new to programming. |
Requirements | Swift is primarily used for developing apps for Apple’s platforms (iOS, macOS, watchOS, and tvOS), so you will need a Mac computer to develop in Swift. You will also need to have Xcode (Apple’s integrated development environment) installed, as well as a basic understanding of how to use it. |
Versatility (Use cases) | ▸ Building native iOS, macOS, watchOS, and tvOS apps ▸ Server-side development using the Vapor framework ▸ Game development using the SpriteKit and SceneKit frameworks ▸ Building machine learning and AI applications using the Core ML framework ▸ Developing augmented reality apps using ARKit |
Developer Salary | $112,657/year (US) ━ €47,000/year (EU) |
Popularity | ❯ StackOverflow Survey: 61.42% approval rate. ❯ PYPL Index: ranked #9. ❯ TIOBE Index: ranked #15. ❯ GitHub: ranked #15. |
Pros | – Easy to learn and use, especially for beginners – Provides fast performance and low memory usage, making it a good choice for developing mobile apps – Works seamlessly with other Apple technologies and frameworks – Provides type inference, which can help reduce the likelihood of errors in code – Open-source and community-driven, which can be helpful for finding support and resources |
Cons | – Limited to Apple platforms, so it may not be suitable for developers looking to build cross-platform apps – Rapid changes and updates to the language and tooling can sometimes make it challenging to keep up-to-date – Lack of backward compatibility, which can require developers to update their code frequently to keep it working with newer versions of Swift |
Useful links | ▪ Website ▪ Documentation ▪ Awesome Swift |
7. Java
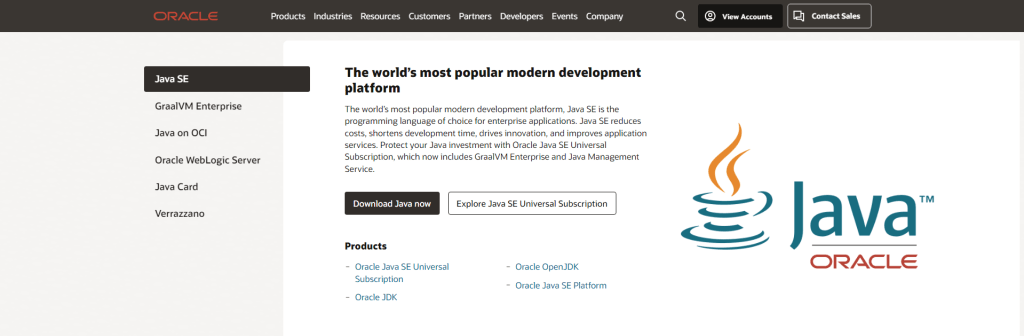
Java is a general-purpose programming language that was first released in 1995 by Sun Microsystems. It quickly gained popularity due to its simplicity, portability, and robustness, and today it is one of the most widely used programming languages in the world.
So what makes Java so special? Well, for starters, it is platform-independent, meaning that code written in Java can run on any device that has a Java Virtual Machine (JVM) installed, regardless of the underlying operating system. This makes Java an ideal language for building cross-platform applications, such as mobile apps and web applications.
Java is also highly secure, with a built-in security model that protects against common vulnerabilities such as buffer overflows and memory leaks. This makes it a popular choice for developing secure applications, such as online banking systems and e-commerce websites.
But what really sets Java apart from other programming languages is its enormous ecosystem of libraries, frameworks, and tools. From the Spring Framework to the Hibernate ORM, from the Eclipse IDE to the Apache Maven build system, Java developers have a wealth of resources at their disposal to help them build robust, scalable, and efficient applications.
Difficulty | Java is considered to have a moderate level of difficulty. It has a strict syntax and requires attention to detail, but it is also a popular language with many resources available to help with learning. |
Learning curve | Java has a moderate learning curve. It can take some time to become proficient, but it is generally considered to be easier to learn than some other popular programming languages like C++. |
Requirements | Java is platform-independent, which means it can be run on a variety of hardware and software systems. However, it does require the Java Development Kit (JDK) to be installed on the computer to develop Java applications. |
Versatility (Use cases) | ▸ Web development (e.g., Java Server Pages, Servlets) ▸ Mobile application development (e.g., Android) ▸ Desktop application development (e.g., Eclipse, NetBeans) ▸ Big data processing (e.g., Hadoop, Apache Spark) ▸ Game development (e.g., Minecraft) |
Developer Salary | $105,128/year (US) ━ €53,500/year (EU) |
Popularity | ❯ StackOverflow Survey: 44.11% approval rate. ❯ PYPL Index: ranked #2. ❯ TIOBE Index: ranked #4. ❯ GitHub: ranked #3. |
Pros | – Platform-independent – Large community and many resources available for learning and support – Robust security features – Excellent for developing enterprise-level applications – Object-oriented programming (OOP) support |
Cons | – Can be verbose and require more code than some other languages – Can have slower performance compared to languages like C++ – Requires the use of a virtual machine which can slow down the application – Memory management can be challenging for beginners – Limited support for low-level programming tasks |
Useful links | ▪ Website ▪ Documentation ▪ Awesome Java |
8. SQL
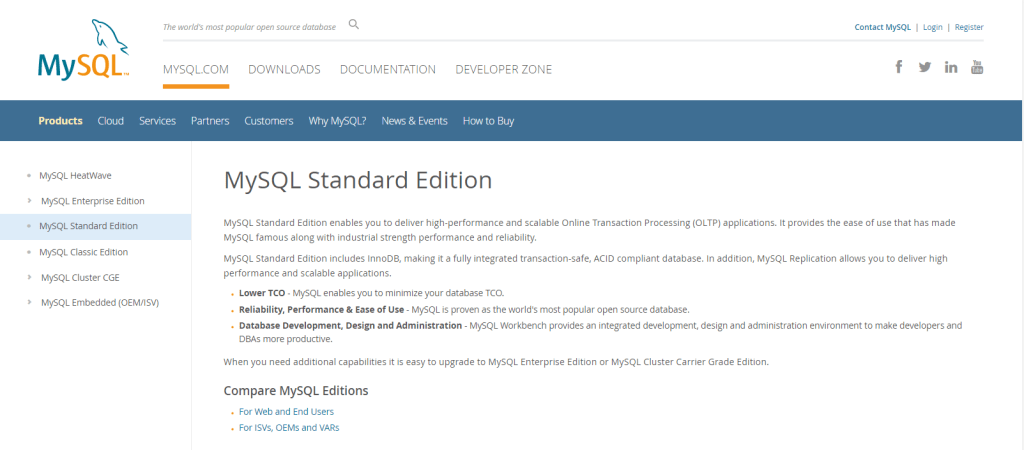
SQL, or Structured Query Language, is a domain-specific programming language that is designed to manage, manipulate, and query data in relational databases. Relational databases are a popular method for organizing data, and SQL is the language that is used to interact with these databases. Popular examples of relational databases include MySQL, Oracle, Microsoft SQL Server, PostgreSQL, and SQLite, among others.
SQL provides a standardized set of commands that can be used to perform a wide range of operations, including data insertion, modification, and deletion, as well as data querying and analysis. Its efficiency and scalability make it well-suited to managing and manipulating large amounts of data, and its use of set-based operations allows it to process data in bulk, making it faster and more efficient than other programming languages that rely on iterative processing.
Difficulty | SQL is considered a relatively easy language to learn, especially compared to other programming languages. The syntax is straightforward, and it uses simple English-like statements to query databases. |
Learning curve | The learning curve for SQL is generally considered to be low, especially for beginners. With some basic knowledge of relational databases, users can learn SQL with ease. |
Requirements | To use SQL, you need a database management system (DBMS) that supports SQL queries. Many popular DBMS options are available, such as MySQL, Oracle, and Microsoft SQL Server. |
Versatility (Use cases) | ▸ Storing and retrieving data for websites and applications ▸ Generating reports and analyzing data for businesses ▸ Managing data for financial institutions and banks ▸ Storing and organizing data for scientific research ▸ Building and maintaining e-commerce platforms |
Developer Salary | $87,255/year (US) ━ €40,000/year (EU) |
Popularity | ❯ StackOverflow Survey: 64.26% approval rate. ❯ PYPL Index: not listed. ❯ TIOBE Index: ranked #9. ❯ GitHub: not listed. |
Pros | – SQL is a standardized language, so code is easily portable between different database – management systems. – SQL is powerful and efficient at managing large amounts of data. – SQL provides a high level of security for data management and storage. |
Cons | – SQL can be limited when it comes to non-relational databases, such as NoSQL databases. – Queries can become complicated and difficult to maintain as the database grows in complexity. – SQL may not be the best choice for high-performance computing tasks or real-time applications. |
Useful links | ▪ Wikipedia ▪ Awesome SQL ▪ SQL Tutorials ▪ MySQL Hosting ▪ MySQL Cheat Sheet |
9. Rust
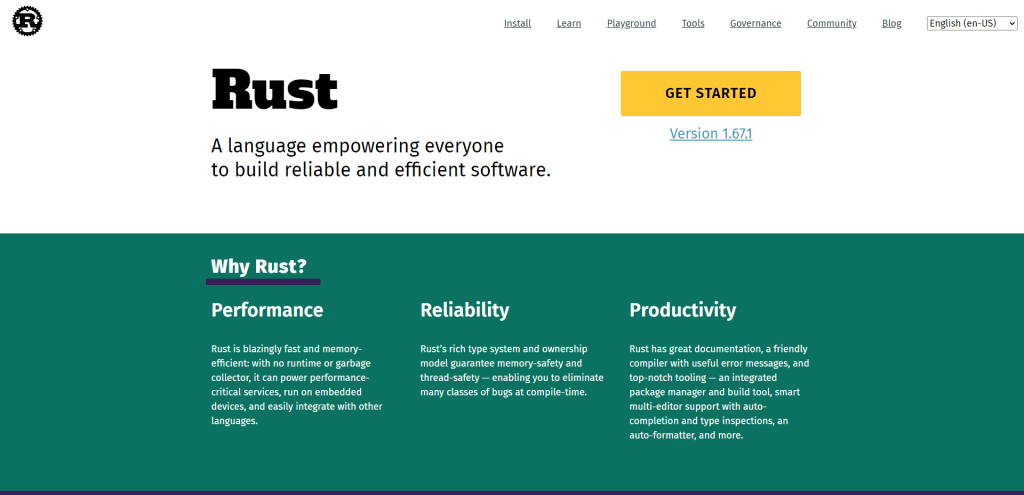
Rust is a fascinating language with a unique and powerful set of features that make it stand out from other programming languages. It was designed with the goal of being fast, safe, and concurrent, and it achieves all three of these objectives in a way that is elegant and efficient.
One of the most notable features of Rust is its memory safety. Rust uses a system of ownership and borrowing to ensure that all memory accesses are safe and secure, without the risk of common issues like buffer overflows, null pointer dereferences, or data races. This alone makes Rust a top choice for system-level programming, where performance and security are critical.
Another key feature of Rust is its expressive type system. Rust’s type system is powerful and flexible, allowing developers to express complex data structures and control flows in a way that is both concise and readable. It also includes support for pattern matching, which is a powerful tool for structuring code and making it more maintainable over time.
Rust is a community-driven language, with a large and growing ecosystem of libraries and tools that make it easier to develop complex applications. There are also many online resources available to learn Rust, including tutorials, books, and videos.
Difficulty | Rust is considered to be a moderately difficult language to learn. It has a steep learning curve compared to some other programming languages, especially for beginners with no prior programming experience. |
Learning curve | Rust requires a good understanding of programming concepts such as memory management, pointers, and ownership. It may take some time to become proficient in these areas, but the official documentation and community resources are very helpful for learning. |
Requirements | Rust requires a compiler to translate code into machine-readable instructions. It can be run on any operating system that supports its compiler and development environment. |
Versatility (Use cases) | ▸ Systems programming (e.g., operating systems, device drivers, web browsers) ▸ Game development ▸ Networking applications ▸ Web development (server-side) ▸ Command-line tools |
Developer Salary | $125,147/year (US) ━ €63,000/year (EU) |
Popularity | ❯ StackOverflow Survey: 84.66% approval rate. ❯ PYPL Index: ranked #11. ❯ TIOBE Index: ranked #20. ❯ GitHub: not listed in the top 20. |
Pros | – Memory safety: Rust’s ownership model prevents many common programming errors such as null pointer dereferences and use-after-free bugs. – Performance: Rust’s emphasis on zero-cost abstractions and control over memory allocation can lead to very performant code. – Community: Rust has a strong and supportive community, which can be helpful for learning and getting help with issues. |
Cons | – Learning curve: Rust’s steep learning curve may be a barrier to entry for some programmers, especially those without prior experience with systems programming. – Syntax: Some programmers find Rust’s syntax to be verbose and difficult to read. – Immaturity: As a relatively new language, Rust’s ecosystem and tooling may not be as fully developed as some other programming languages. |
Useful links | ▪ Website ▪ Documentation ▪ Awesome Rust |
10. Go
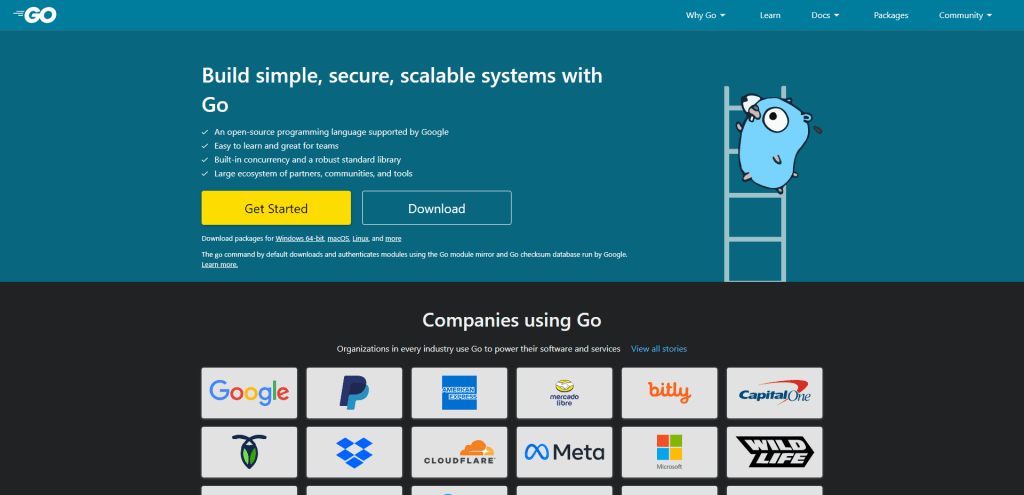
Go is a modern programming language created by Google in 2009 with the goal of improving the efficiency and scalability of software development. It was designed to be simple and straightforward, while also offering the performance of a low-level language like C or C++.
Go is a compiled, statically-typed language that is designed to be simple and easy to use, yet incredibly powerful. It features a concise syntax and a lightweight, fast and efficient runtime, making it ideal for building large-scale, high-performance applications.
One of the most striking features of Go is its built-in support for concurrency, which allows for the creation of lightweight, independently executing processes that can communicate with one another through channels. This makes it easy to write programs that can take full advantage of modern multi-core processors, without the need for complex locking mechanisms or other synchronization primitives.
In addition to its concurrency features, Go also has strong support for networking and web development. Its standard library includes a robust set of tools for building HTTP servers and clients, as well as handling databases, encryption, and other common tasks.
But what sets Go apart from other programming languages is not just its technical capabilities. It is also an incredibly community-driven language, with a large and passionate developer community that is dedicated to helping each other learn and grow.
Difficulty | Go is considered a relatively easy language to learn and use, even for beginners with no prior programming experience. |
Learning curve | The learning curve for Go is generally considered to be low to moderate. The language is designed to be simple and easy to pick up, with a focus on practicality and efficiency. |
Requirements | Go can be used on a wide range of hardware and software platforms, including Windows, macOS, Linux, and others. It can be compiled on different architectures and supports cross-compiling, making it easy to build applications for different platforms. |
Versatility (Use cases) | ▸ Network programming ▸ Cloud infrastructure development ▸ Web development ▸ System programming ▸ Machine learning |
Developer Salary | $121,764/year (US) ━ €62,000/year (EU) |
Popularity | ❯ StackOverflow Survey: 62.45% approval rate. ❯ PYPL Index: ranked #12. ❯ TIOBE Index: ranked #11. ❯ GitHub: ranked #14. |
Pros | – Easy-to-learn syntax and standard library – Fast execution times and efficient memory management – Built-in concurrency support – Cross-platform compatibility – Strong typing and error checking – Growing community and extensive documentation |
Cons | – Limited support for object-oriented programming (OOP) features – Not as mature as some other programming languages – Limited third-party library support compared to more popular languages like Python and Java. |
Useful links | ▪ Website ▪ Documentation ▪ Awesome Go |
Summary
If you’ve gotten this far, you’ve made it through more than 6,500 words of content; phew! I’ll be monitoring the feedback about this article. Still, as far as my research went – I don’t think you are going to find a more comprehensive overview of the currently most popular programming languages. That said, you can always ping me on Twitter or send me an email with suggestions.