I love experimenting with various animation effects, particularly those that are simple and can be implemented with CSS. But, if we look at the broader picture – CSS animations will only take you that far. And, if you want to create complex and interactive user experiences, you’ll have to switch to JavaScript sooner or later.
The main advantage of using JavaScript for animation effects is that you can control more of the animation logic. This includes the fluidity of transitions, controlling DOM state and response, and using 2D and 3D graphics thanks to WebGL.
The types of JavaScript animation libraries
So, because JS animation libraries come in many shapes and forms, it really helps to narrow down the specific area of focus. Many engines and frameworks aren’t just used for front-end stuff, but also used to build games, and create other interactive content.
For this specific roundup, my focus is on libraries that are most commonly used in front-end development, either on their own or together with any of the current frameworks.
In due time, I’ll consider adding more libraries to this list that are smaller in scale but still provide meaningful ways to add interactive animations to your projects.
Each library has links to its website and GitHub page. I’ve also added a CodePen example that you can run from this page and additional resources – which are either tutorials or video guides.
Three.js
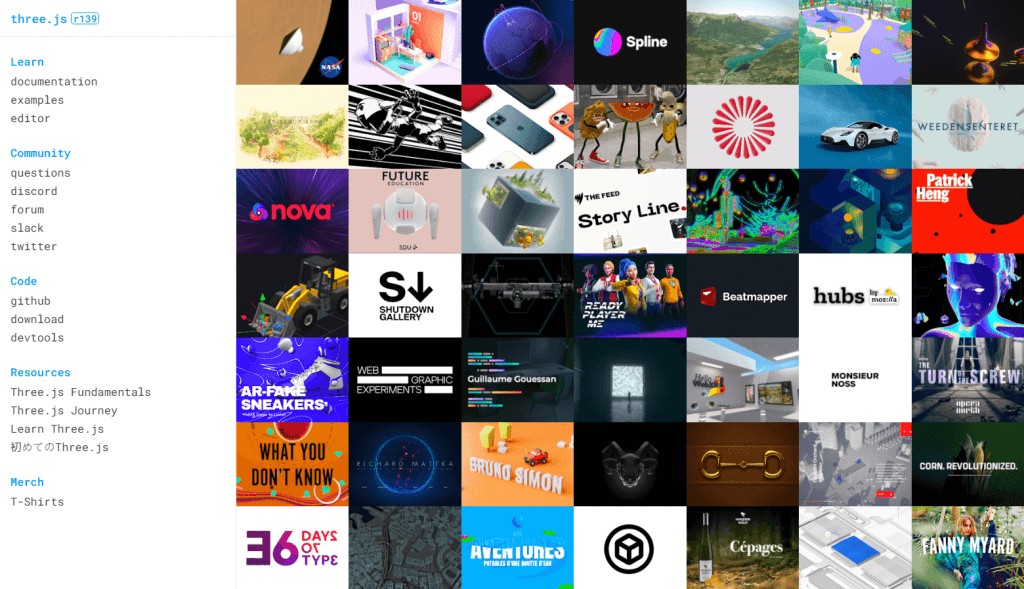
Three.js is the go-to library for creating 3D animated effects that you use in creative website development. Three.js removes the need for developers to learn about WebGL, and can instead focus on building interactive 3D effects without the complexity.
On the high end, Three.js is used to create interactive virtual experiences like Mozilla Hubs. The library is also often utilized to create immersive landing page experiences. World-class publishers and editorials have used Three.js for years to build data-based pages with dynamic updates.
If you visit the homepage, many highlighted projects are listed, which should give you plenty of inspiration/clarity about how this library is used in everyday environments.
Three.js Example Animation
See the Pen Mesh Line Waves Background – THREE.js by CP Designer (@cpandya) on CodePen.
Anime.js
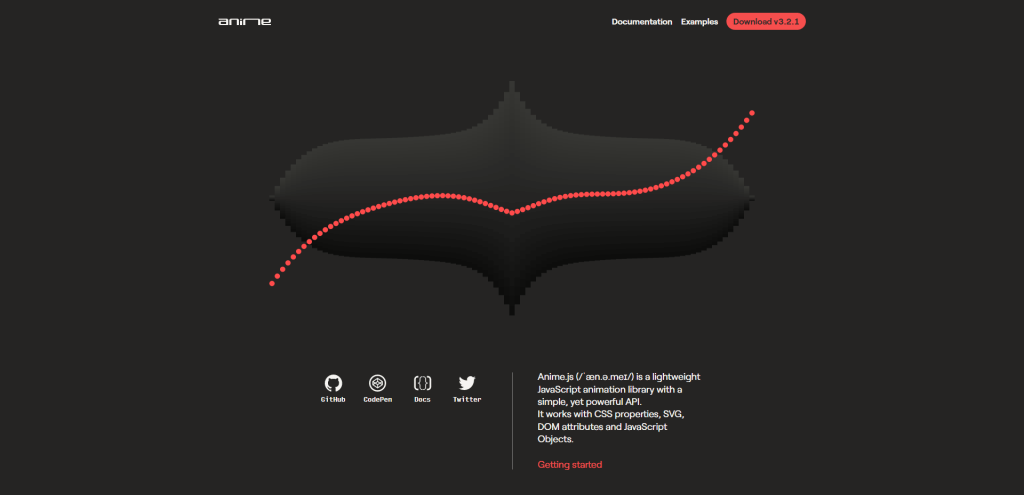
Anime.js from Julian Garnier is probably the 2nd best-known library for integrating animations into web-based projects. Its popularity stems from having in-built tooling to help accelerate the process of animating CSS, SVG, and DOM elements.
For example, you can target specific CSS selectors and then apply refined animation logic through JavaScript, as opposed to writing the @keyframes yourself.
anime({
targets: '.staggering-axis-grid-demo .el',
translateX: anime.stagger(10, {grid: [14, 5], from: 'center', axis: 'x'}),
translateY: anime.stagger(10, {grid: [14, 5], from: 'center', axis: 'y'}),
rotateZ: anime.stagger([0, 90], {grid: [14, 5], from: 'center', axis: 'x'}),
delay: anime.stagger(200, {grid: [14, 5], from: 'center'}),
easing: 'easeInOutQuad'
});
Just with the above snippet alone, you can make an effect that looks like this:
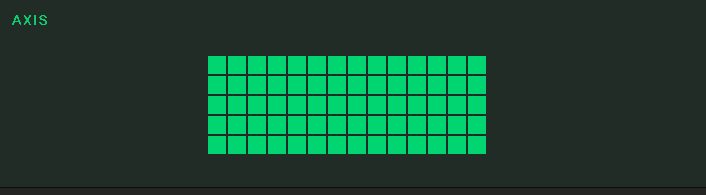
If you were to do this with CSS – you would need to select each individual grid block and write separate logic for it. Not only is that impractical, but it’s also a lot of work. On the Anime.js docs page, you’ll find plenty of similar examples. And, the demo below goes a step further to showcase how this library can be used in modern front-end development.
Anime.js Example Animation
See the Pen anime.js logo animation by Julian Garnier (@juliangarnier) on CodePen.
Popmotion
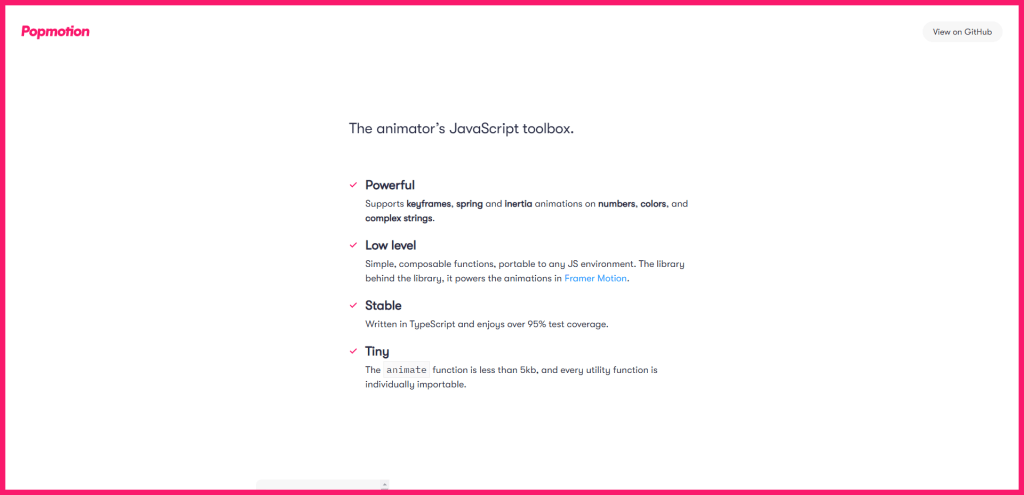
Popmotion is a low-level animation library written in TypeScript. The library also powers the popular Framer Motion (we have it listed in this article) library used in React projects. Being unopinionated, you can use Popmotion to integrate custom effects by writing additional functions you wish to use.
As the name implies, Popmotion is particularly good for animating user interface elements through the use of various motion-based animations. This includes effects like easing, springs, keyframes, and more intricate transition effects. The example below does a good job of showcasing the practical application of animation effects for component elements.
Popmotion Example Animation
See the Pen Popmotion demo’s by Arden (@aderaaij) on CodePen.
mo.js
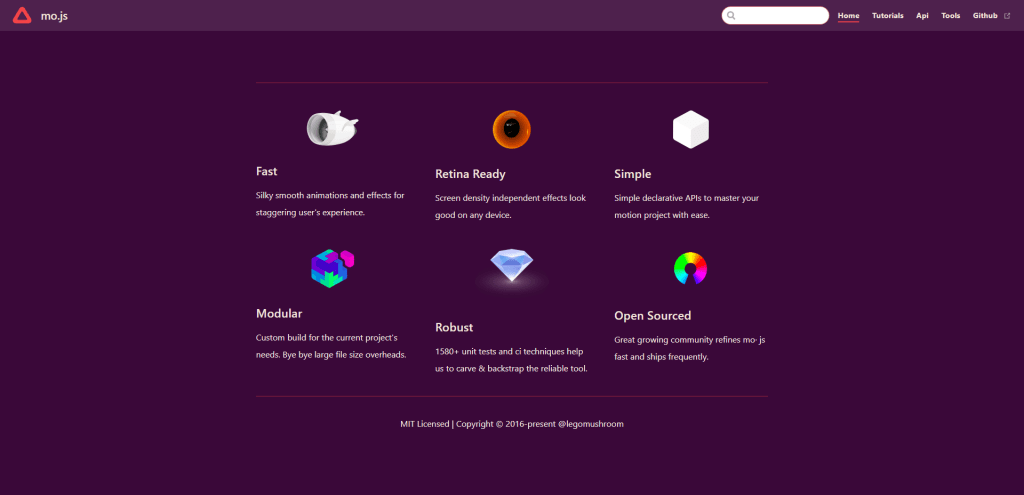
Mo.js is focused on motion graphics and excels in providing a simple code structure that you can implement in self-contained projects and with frameworks such as React.
And, because Mo.js ships with its own declarative API – you get to control each step of the animation. This includes defining the logic for what you wish to achieve and how you will get there. The library includes pre-made components and examples which are tailored for visually rich user experiences.
Here is a sample snippet:
const travelCircleExpand = new mojs.Shape({
fill: COLORS.BLACK,
radius: 126,
scale: { .1: 1 },
opacity: { 0 : 1 },
easing: 'cubic.out',
duration: 400,
isForce3d: true,
isTimelineLess: true,
});
As you can see, a lot of the logic is pre-defined. So, rather than coming up with your own ideas, you can take note of the provided properties, and build your way up. The tutorial section in the docs has quite a few examples of practical applications, particularly effects that add minuscule yet meaningful animations to everyday web page elements.
mo.js Example Animation
See the Pen Link Hover Effects w/ mo.js by Mike Quinn (@mprquinn) on CodePen.
p5.js
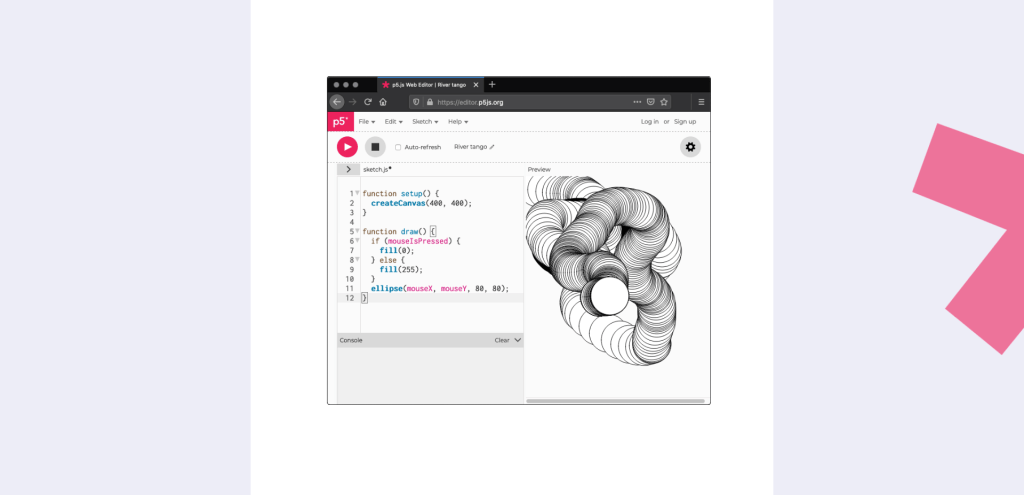
p5.js is the JavaScript implementation of Processing – a separate “language” intended for use by visual artists. Unlike some of the examples we have seen so far, p5.js is a universal animation library, providing solutions for practical applications and more robust and complex projects. This includes full-on support for 2D & 2D effects.
As far as using p5.js in website projects go, the library opens up an avenue for in-depth creativity. For example, you can quickly bootstrap effects like a smokescreen, animated trees, and data-based landing pages which users can interact with.
You do have to do all of the drawings yourself, but with the number of resources available for p5.js – I think you’ll find that it’s not so difficult to get started. And, it goes without saying, that the community behind both of these projects is very involved.
p5.js Example Animation
See the Pen P5.JS Twist and Turn. by Sdsmnc (@supastrocat) on CodePen.
Motion
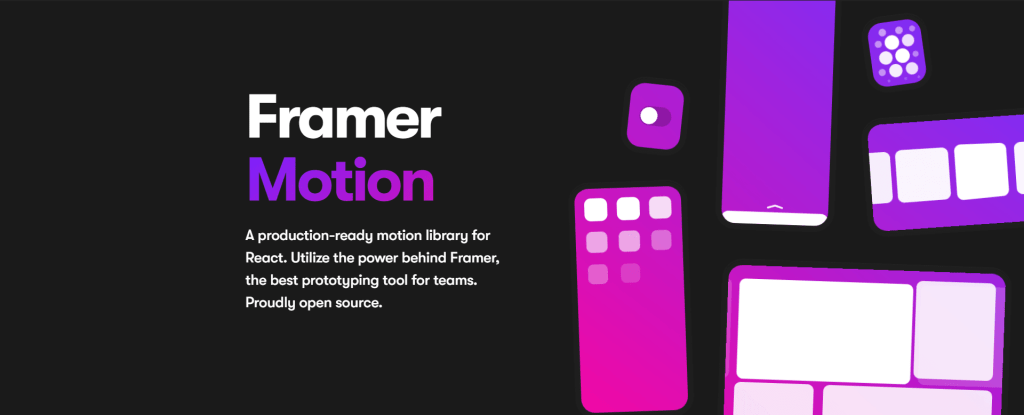
React is so popular that it only makes sense it would have its own animation library. Framer Motion comes with a pre-built API that let’s React developers simplify the process of building animated components. It also alleviates some of the hurdles of needing to learn CSS and its independent animation properties. And it’s quite easy to work with.
An example snippet:
import { motion } from "framer-motion"
export const MyComponent = () => (
<motion.div
animate={{ rotate: 360 }}
transition={{ duration: 2 }}
/>
)
Animations in Framer are defined by the motion
& animate
properties. This lets you select entire components and their inner elements, which you can then enrich with your custom animation logic. The Smashing article from Nefe Emadamerho-Atori in the resources section is a great starting point to see how Framer Motion works.
Motion Example Animation
See the Pen Laser Sight Button by codebro (@codebro) on CodePen.
GSAP
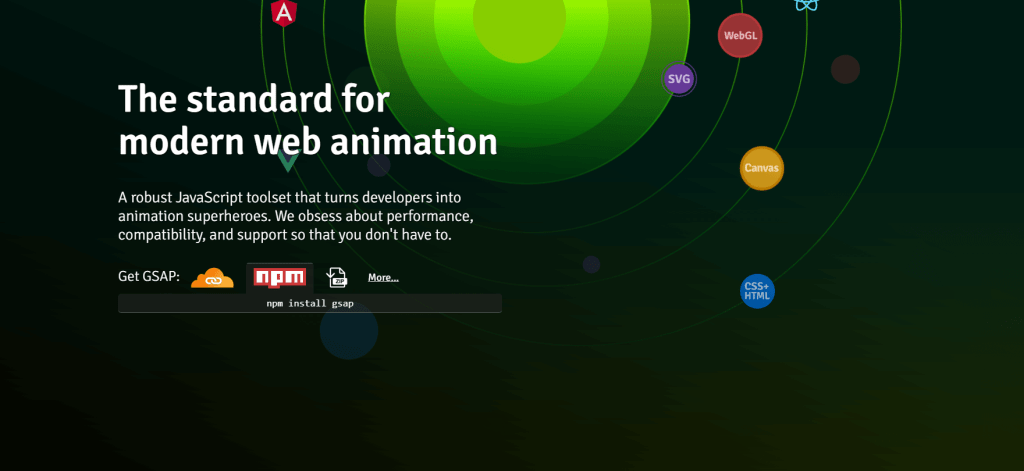
I’ve been seeing GSAP art being shared on Twitter quite a lot recently. But it doesn’t come as a surprise. Many of the animations and dynamic effects we see in creative projects have been made possible thanks to the robust GSAP engine.
Not only is the library optimized for performance, but it’s also highly compatible with your favorite technologies. That includes frameworks like React & Vue and libraries like jQuery, with additional support for mobile and dated web browsers.
Because GSAP can query and animate practically any web element (from CSS to Canvas to DOM objects), you can use it for something as simple as a spinner effect, or go all out and build truly dynamic website experiences.
The Showcase section has hundreds of example projects developers have worked on. Check it out for inspiration and to see what is possible with this library.
GSAP Example Animation
See the Pen Hulu Originals Intro by Hyperplexed (@Hyperplexed) on CodePen.
Paper.js
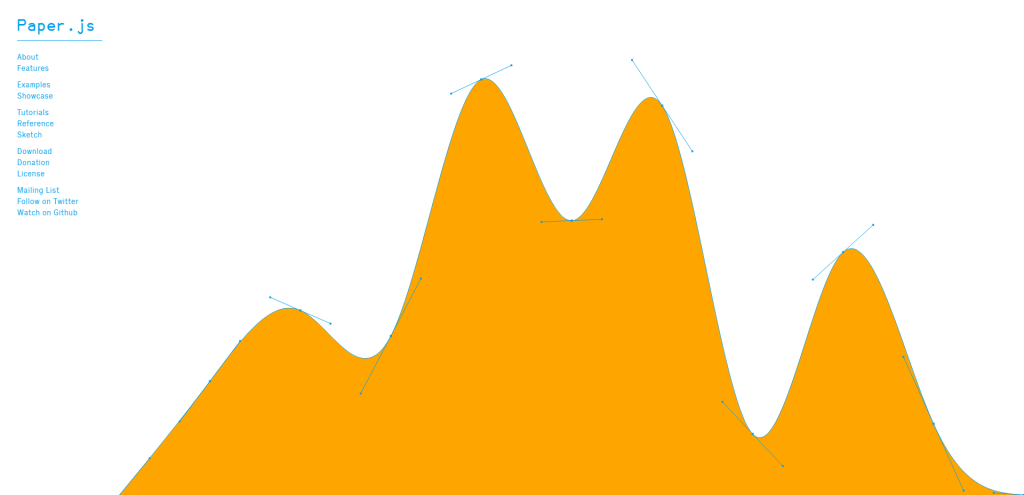
Paper.js is an animation library with a strict focus on animating vector graphics. This approach lets you do not only static effects, but also interactive dynamic experiences. In particular, Paper.js is popular among animation projects where users can drag objects, rearrange them, and provide custom input.
Objects can be categorized through layers, each with a custom animation specification. This comes in handy when you work on complex structures, letting you remove/disable certain layers unless requested by the user.
Paper.js Example Animation
See the Pen Filling Glasses – Paper.js by Fiorald Ismaili (@Fiorald) on CodePen.
Web Animations
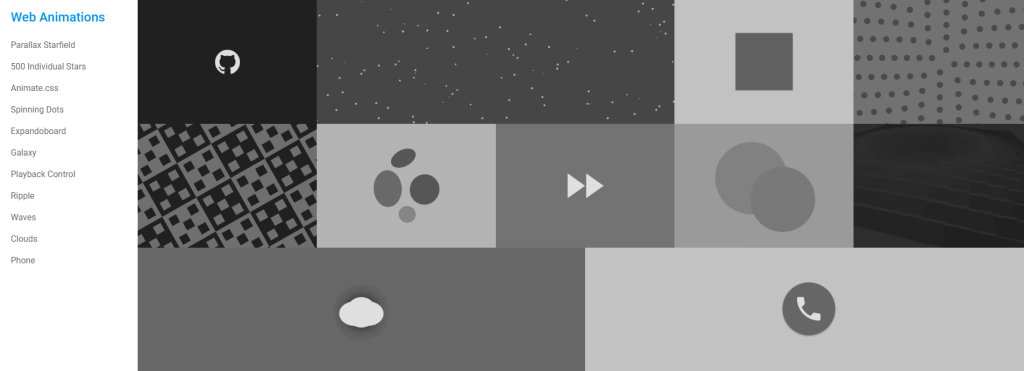
This library is a direct JavaScript port of the Web Animation API. The library integrates directly with the Element.animate()
specification, letting you use animation features typically written using CSS logic. The authors explained it by saying:
“A JavaScript implementation of the Web Animations API that provides Web Animation features in browsers that do not support it natively. The polyfill falls back to the native implementation when one is available.”
Web Animations Example Animation
See the Pen Imperative Animations by Sam Thorogood (@samthor) on CodePen.
Proton
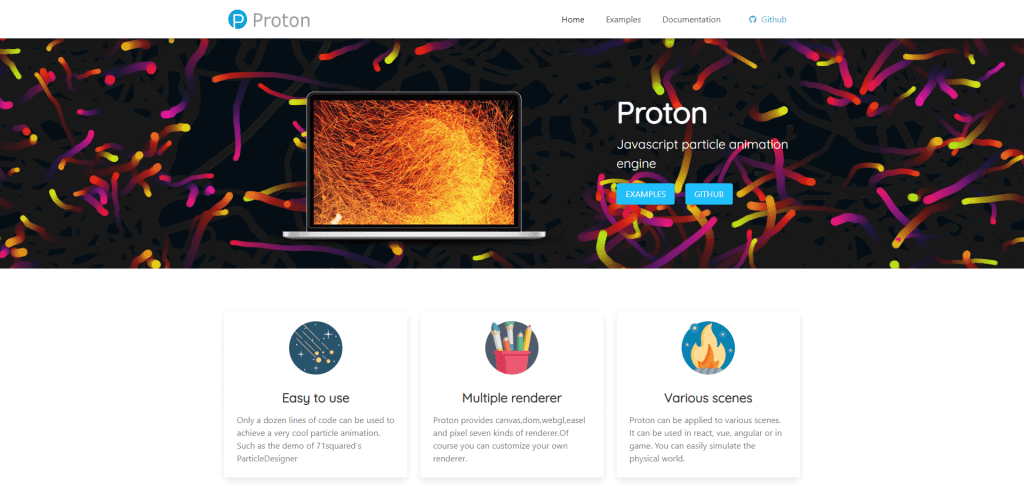
Particle effects are definitely up there in modern web design trends. Not only do designers implement them for background effects, but also for interesting transitions, and even presentations for creative projects. The Proton library is tailored specifically to the needs of quickly scaling creative particle effects.
You can do things like build spark effects, and collision-based interactions, but also transform text into new and exciting animation experiences.
Proton Example Animation
See the Pen my-emitter by matsu7089 (@matsu7089) on CodePen.
Summary
The nice thing about many of these libraries is that they have been around for a while. As such, it’s relatively easy to find examples and in-depth guides on using a particular library in a specific context.
Above all, it helps to know what kind of a goal you’re trying to achieve, particularly if you’re going to be working on animations that implement real-time data or user-based input.
In other words, there is no such thing as best. Each library has its own strengths and weaknesses. I’d probably go with GSAP because it’s nicely optimized for use in almost any front-end project imaginable.
But if I am doing a project that’s less ambitious, a library like Popmotion is plenty.